WGU D286 OA Study Guide I I - 2025 | Java Basics: Arrays, Setters, Getters, and Loops📖
Welcome to the exciting world of Java programming, where you’re about to learn three foundational pillars that every coder should master: Classes: Arrays and Structures, operators: Setters & Getters, and Loops. It may help to consider these concepts as your practical tools–you might be working on constructing an entire city’s worth of code, and reach for these tools, or, you may be attempting to remove a small scratch on the project and you find yourself using these tools.
- Java Arrays and ArrayLists: Arrays store fixed-size collections of elements, while ArrayLists are dynamic, allowing resizing and built-in methods for easier manipulation.
- Java Setters and Getters: Setters allow modifying private variables, while getters retrieve their values, ensuring encapsulation and controlled data access in Java classes.
- Java For Loops and While Loops: For loops are ideal for fixed iterations, while while loops execute repeatedly based on a condition, providing flexibility in loop control.
Throughout this article, you’ll dive into practical examples, unravel common mistakes, and discover best practices. By the end, you’ll be ready to tackle any Java problem the WGU D286 module throws your way. So, roll up your sleeves, grab your favorite beverage, and let’s decode the mysteries of Java together!
How to Use This Guide for the WGU D286 OA Exam?📖
The D286 Java Fundamentals OA exam at WGU evaluates your understanding of Java programming concepts, methods, and constructors. This guide simplifies the key concepts of Java constructor and overloaded constructor, Java AWS Shield & Guard Duty, and using methods and calling them to help you grasp the topics tested in the exam.
We also provide exam-style questions and practical applications to ensure you’re fully prepared for the questions on the WGU D286 OA exam.
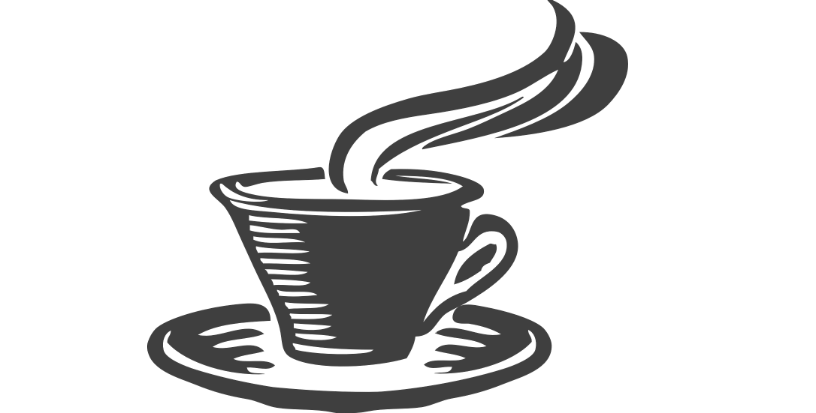
Java Arrays and ArrayLists For D286 OA📝
Java programming often involves handling collections of data, and two common tools for this are Arrays and ArrayLists. While both serve the purpose of storing multiple elements, they are different in terms of flexibility, structure, and functionality. In this section, we will explore the basics of Arrays and ArrayLists, guiding you step-by-step to ensure a solid understanding. This knowledge will be especially helpful for your success in the WGU D286 module.
Arrays in Java
What is an Array?
Suppose you have a line of compact storage, each labeled numerically in order to allow storage of several similar items—this is an Array. They help you to store several related values under one name and they are easy to manage as well. Java Array is an aggregate of several elements in Java, grouped under a single variable name, and all the elements should be of the same type, such as numbers, characters, or any objects. This implies that one can arrange data methodically since each component within the array should be a member of a particular cell that can be indexed uniquely.
Declaring and Initializing Arrays
To create an Array, you need to declare its type and size. Let’s break it down:

Accessing and Modifying Elements
In an Array, each element is accessed using an index, starting at 0. Here’s how it works:

Useful Properties and Operations
- Length: Use arrayName.length to find the number of elements.
Iterating through an Array:
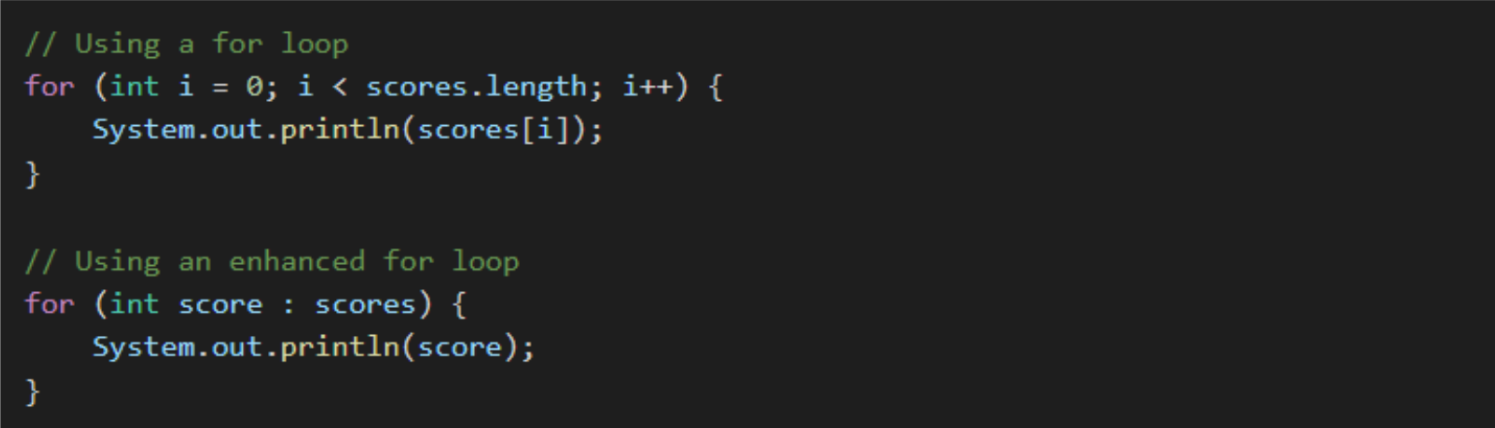
Limitations of Arrays
While Arrays are efficient and provide fast access to elements, they come with some constraints that might limit their usability in certain scenarios:
- Fixed-size: Once an Array is created, its size cannot be changed.
- Lack of built-in methods for dynamic operations like adding or removing elements.
ArrayLists in Java
What is an ArrayList?
I hold an ArrayList as a rather dynamic list that could have more or fewer items depending on the needs, it is like a bag that can grow large enough to contain all your items. Unlike Arrays, ArrayLists can be modified by either adding more elements or even by reducing the number of elements in it as seen below. The ability of these structures to be flexible allows them to be utilized in a situation in which the number of items is unknown. Also, there are classes of Java Collections Framework for Java that offer a perfect set of tools and methods for the elaboration of collections containing the necessary amount of data.
Declaring and Initializing ArrayLists
To use an ArrayList, you must import it from the java.util package. Here’s how to declare and initialize one:
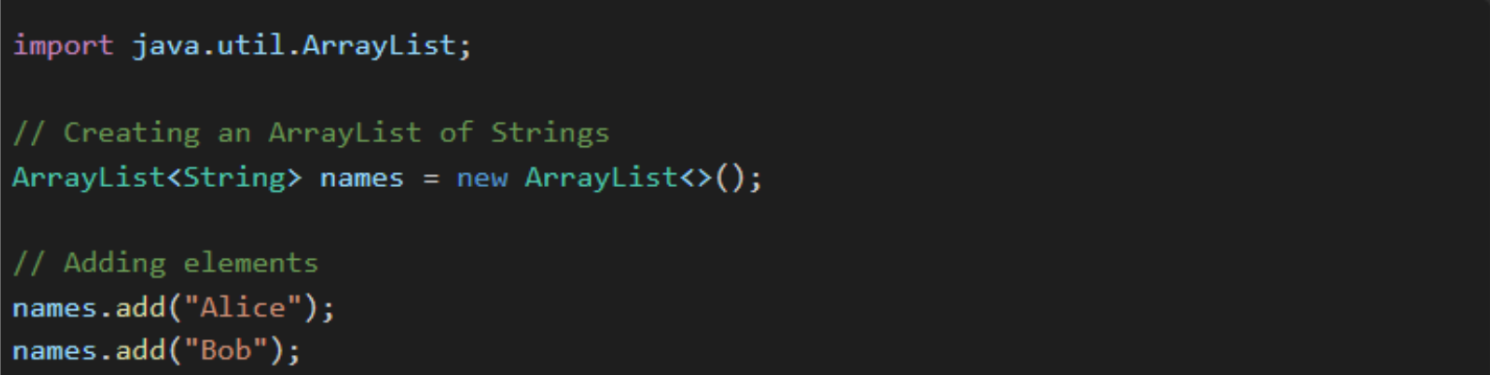
Accessing and Modifying Elements
Similar to Arrays, you can access and modify elements in an ArrayList using indices.

Dynamic Operations
ArrayLists provide a variety of methods to simplify tasks:
- Adding elements: add(element)
- Removing elements: remove(index) or remove(object)
- Checking size: size()
- Iterating through elements:

Converting Between Arrays and ArrayLists
Sometimes, you may need to convert data between Arrays and ArrayLists. Here’s how:
Array to ArrayList:

ArrayList to Array:

Practical Use Cases
- Arrays:
- Fixed data like grades or coordinates.
- Performance-critical applications requiring primitive data types.
- ArrayLists:
- Dynamic lists such as user input data or inventory.
- Applications requiring frequent additions or deletions of elements.
Common Pitfalls and Best Practices
- Index Out of Bounds:
- This happens when you try to access an element at an index that doesn’t exist in the Array or ArrayList.
Example:

- Best Practice: Always check the size of the collection using arrayName.length or arrayList.size() before accessing elements.
2. Use Enhanced Loops:
- Enhanced loops (also called “for-each” loops) are simpler and reduce the chances of making errors related to indexing.
Example:

- Best Practice: Use enhanced loops whenever you don’t need the index explicitly.
3. Choose the Right Tool:
- Arrays are better suited for fixed-size data where the number of elements is known in advance.
- ArrayLists are more appropriate for scenarios where the size of the data changes dynamically.
- Best Practice: Consider the use case. If you need dynamic resizing and built-in methods like add() or remove(), go for ArrayLists. For static and performance-critical data, choose Arrays.
4. Index Out of Bounds: Ensure you don’t access indices outside the valid range.
5. Use Enhanced Loops: Simplify iteration for better readability.
6. Choose the Right Tool: Use Arrays for fixed-size data and ArrayLists for dynamic collections.
Importance For D286 OA
Understanding the differences between Arrays and ArrayLists is essential for Java programming. Arrays are great for fixed-size collections, while ArrayLists provide flexibility for dynamic data. Both tools are powerful in their own ways and play a crucial role in mastering Java fundamentals. As you prepare for the WGU D286 module, practice using these data structures in simple programs to build confidence.
Stay tuned for more insights to help you succeed with WGU D286 OA questions and beyond!
Java Setters and Getters For D286 OA📝
When working with Java, encapsulation is a core concept of object-oriented programming. It involves bundling the data (variables) and the code (methods) that manipulates the data into a single unit—a class. To ensure the integrity of the data, we make variables private and provide controlled access to them using methods called setters and getters. In this section, we’ll explore how setters and getters work, why they are important, and how to use them effectively in your programs. Understanding these concepts will give you a strong foundation for the WGU D286 module.
What Are Setters and Getters?
Definition
- Getters: Methods that retrieve the value of a private field.
- Setters: Methods that set or update the value of a private field.
Purpose
Setters and getters promote encapsulation, a key principle of Java programming. By controlling access to fields, they:
- Protect the internal state of the object.
- Allow for validation and processing before data is stored or retrieved.
Example
Here’s a simple example to illustrate:
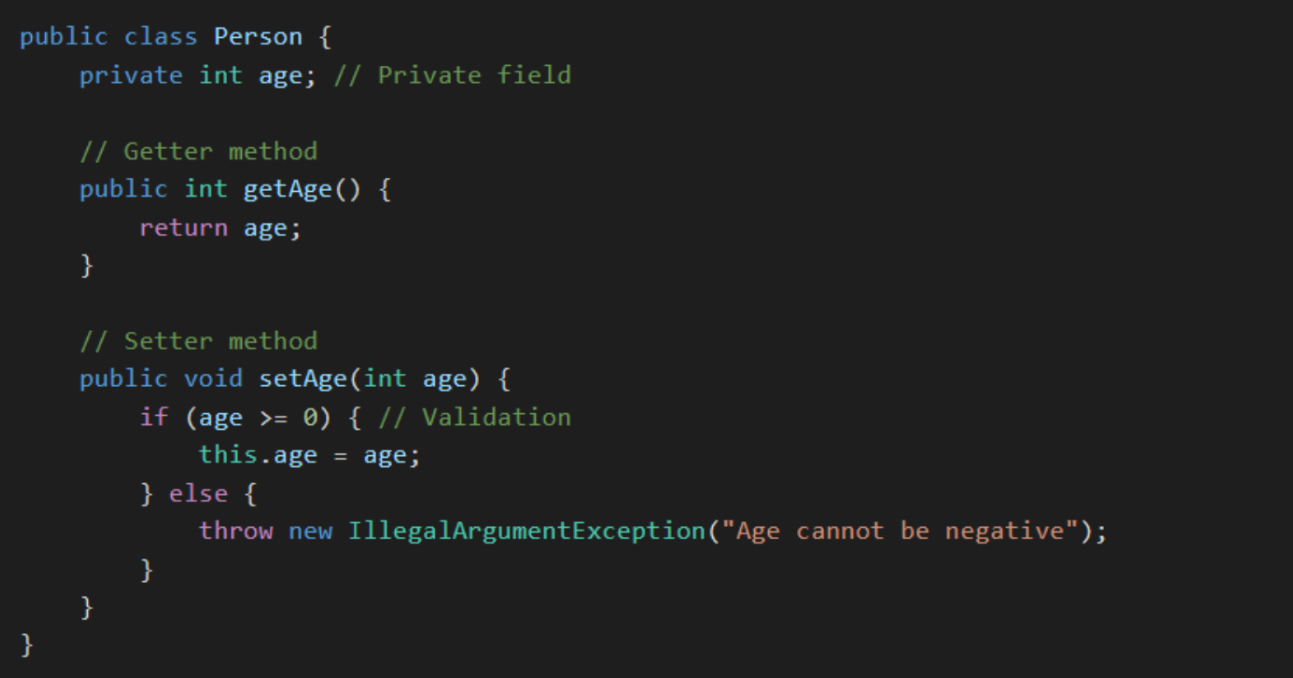
In this example, the age field is private, but it can be accessed and modified safely through the getAge and setAge methods.
Key Concepts
Encapsulation
Encapsulation ensures that an object’s internal state is hidden from the outside world. By keeping fields private and using setters and getters for access, you:
- Prevent unintended changes to the data.
- Enable validation and control over how fields are modified.
Naming Conventions
Setters and getters follow specific naming patterns:
- Getters: getFieldName() (e.g., getName() for a field named name).
- Setters: setFieldName() (e.g., setName(String name)).
- For boolean fields, the getter can use isFieldName() (e.g., isAvailable()).
Input Validation in Setters
Setters can include logic to ensure that only valid data is accepted. For example:
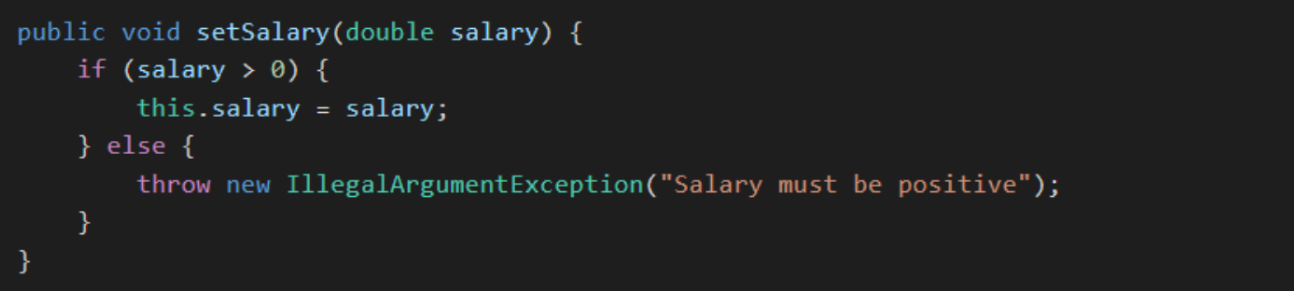
Advanced Features
Data Processing in Getters and Setters
Sometimes, you might need to process data within these methods. For example:
- Getter: Format the value before returning it.
- Setter: Split a full name into first and last names.
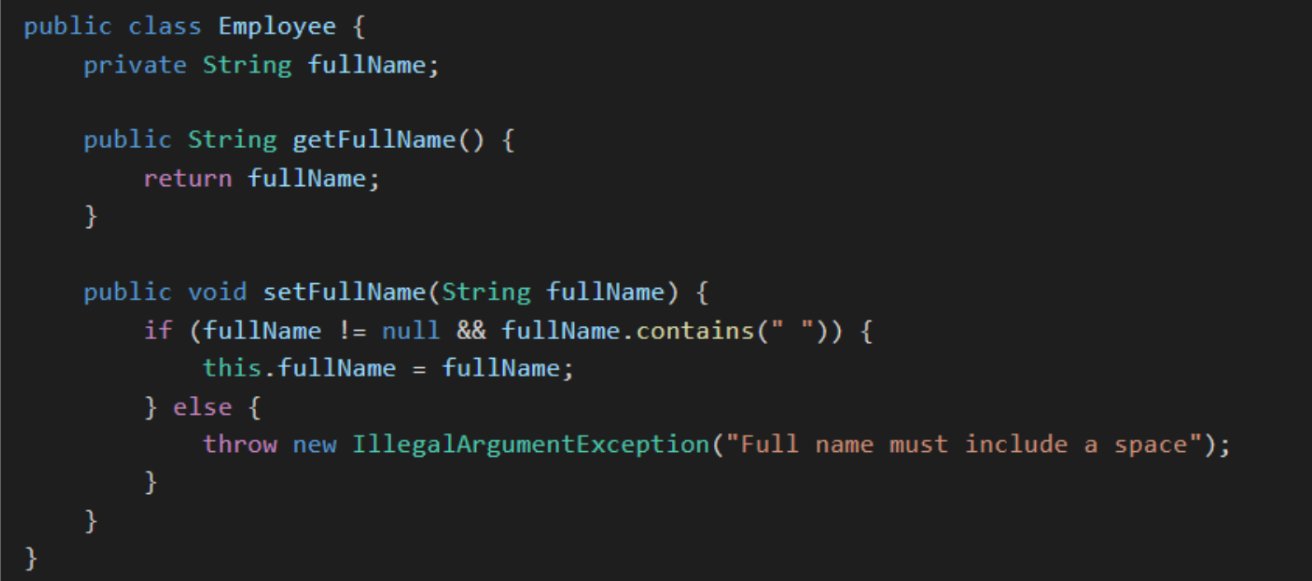
Defensive Copies
For fields that hold mutable objects (like arrays or collections), getters should return a copy to avoid external modifications:
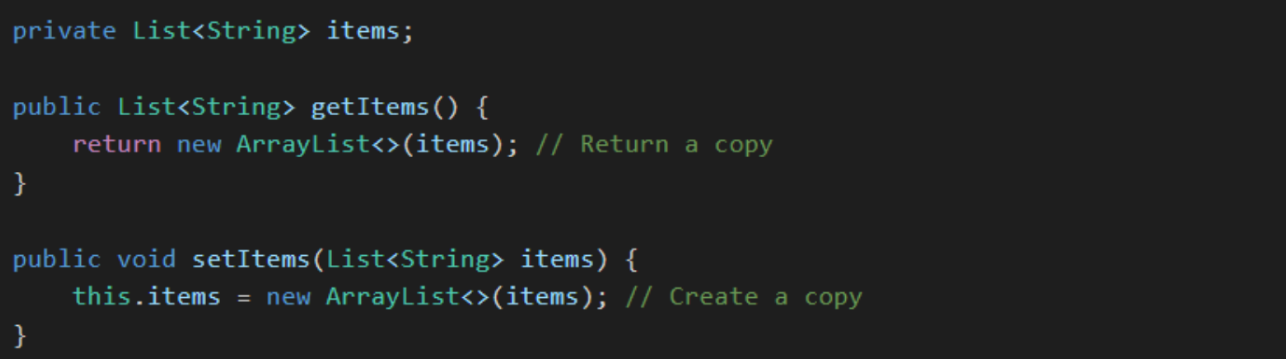
Overloading Setters
Setters can be overloaded to handle different input formats. For instance:
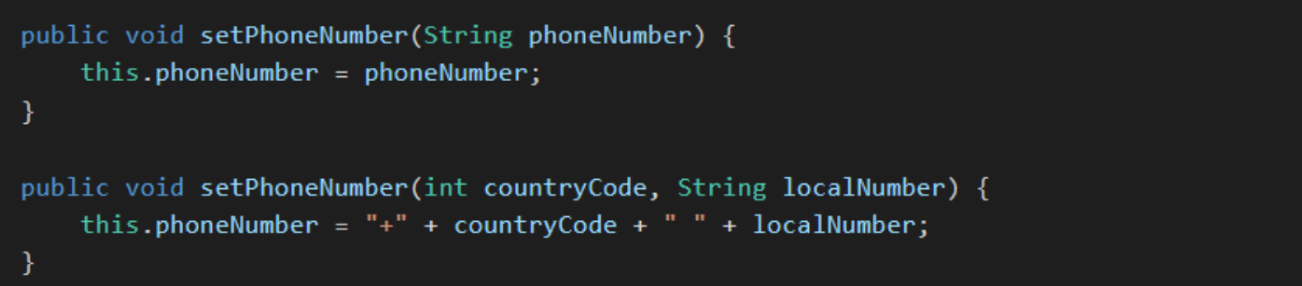
Practical Applications
- Frameworks: Getters and setters are essential in frameworks like JavaBeans, where they enable property management.
- Data Validation: Use setters to enforce constraints, such as non-negative values or valid formats.
- Hands-on Practice: Create a Student class with fields like name and grade, then implement and test corresponding getters and setters.
Common Mistakes and Best Practices
- Mistake: Forgetting to validate input in setters.
- Solution: Always include checks to ensure data integrity.
- Solution: Always include checks to ensure data integrity.
- Mistake: Returning mutable objects directly from getters.
- Solution: Use defensive copies to protect the internal state.
- Solution: Use defensive copies to protect the internal state.
- Mistake: Overusing getters and setters for every field.
- Solution: Only create these methods when access control or additional logic is needed.
- Solution: Only create these methods when access control or additional logic is needed.
- Best Practice: Use IDE tools like Eclipse or IntelliJ IDEA to auto-generate getters and setters, saving time and reducing errors.
Importance For D286 OA
Setters and getters are fundamental to encapsulation in Java, ensuring that an object’s internal state remains protected while providing controlled access. By following best practices—such as including validation in setters and using defensive copies—you can write robust and maintainable code. These methods are integral to mastering object-oriented programming and succeeding in the WGU D286 module. Practice creating classes with properly implemented setters and getters to solidify your understanding and prepare for WGU D286 OA questions!
Tired of reading blog articles?
Let’s Watch Our Free WGU D286 Practice Questions Video Below!
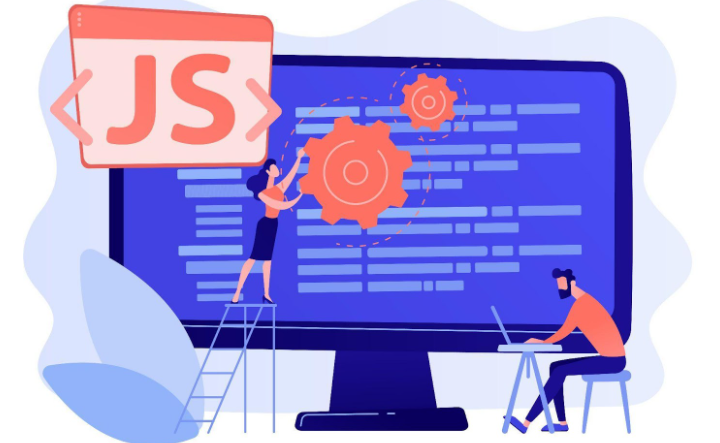
Java For Loops and While Loops For D286 OA📝
In programming, repeating tasks efficiently is crucial. This is where loops come in. Java provides several looping structures that allow developers to execute a block of code multiple times based on a condition. Two fundamental types of loops in Java are for loops and while loops. In this section, we will break down these loop structures step by step to ensure you understand how and when to use them. Mastering these concepts is essential for success in the WGU D286 module.
For Loops
What is a For Loop?
A for loop is a control structure that is used when the number of iterations is known beforehand. It repeats a block of code a specific number of times.
Syntax
The syntax of a for loop consists of three parts inside the parentheses:

Components
- Initialization: This is where you declare and initialize a loop control variable.
- Condition: The loop runs as long as this condition evaluates to true.
- Update: This updates the loop control variable after each iteration.
Example
Here’s a basic example:

Explanation:
- int i = 1: Starts the loop control variable at 1.
- i <= 5: Runs the loop while i is less than or equal to 5.
- i++: Increments i by 1 after each iteration.
Use Cases
- Iterating over arrays or collections.
- Performing repetitive calculations like summing numbers.
While Loops
What is a While Loop?
A while loop is used when the number of iterations is not known beforehand. It keeps executing the code block as long as the specified condition evaluates to true.
Syntax
The syntax of a while loop is simpler compared to a for loop:

Example
Let’s look at an example:

Explanation:
- int count = 5: Initializes the control variable.
- count > 0: The loop continues as long as count is greater than 0.
- count–: Decrements count by 1 after each iteration.
Use Cases
- Repeating actions until a condition is met, such as validating user input.
- Reading data until the end of a file.
Do-While Loops
What is a Do-While Loop?
The do-while loop is similar to the while loop but guarantees that the code block is executed at least once, even if the condition is initially false.
Syntax

Example

Explanation:
- The code inside the do block executes at least once, prompting the user for input.
- The loop continues until a positive number is entered.
Nested Loops
You can place one loop inside another, known as nested loops. These are especially useful for working with multi-dimensional arrays.
Example

Loop Control Statements
Break Statement
Exits the loop immediately, regardless of the condition.

Continue Statement
Skips the current iteration and moves to the next one.

Common Errors and Debugging Techniques
- Infinite Loops:
- Occurs when the loop condition is never met.
- Debug by adding print statements or using an IDE debugger.
- Off-by-One Errors:
- Happens when the loop runs one iteration too many or too few.
- Carefully check your loop conditions.
Practical Exercises
- Print Multiplication Table:
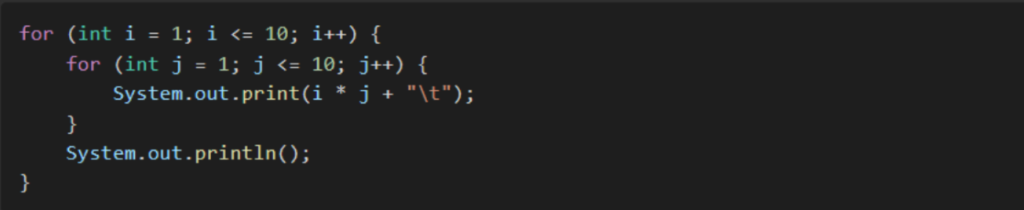
2. Calculate Factorial:

Importance For D286 OA
Understanding loops is essential for writing efficient and reusable code in Java. Whether using for loops for predictable iterations or while loops for conditional repetition, these constructs allow you to automate repetitive tasks effectively. Practice using loops in different scenarios to build your confidence and prepare for the WGU D286 OA questions. This knowledge will greatly enhance your programming skills and set a strong foundation for advanced topics.
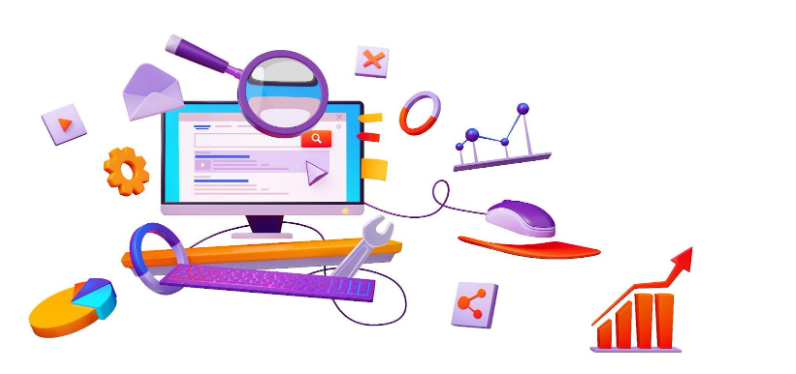
Wrapping Up: A Final Note for WGU D286 Success📄
Congratulations on making it through this journey into Java’s core concepts! We’ve explored Arrays, Setters and Getters, and Loops—three essential tools in your programming toolkit. By now, you should feel more confident in understanding how to organize data, safeguard it with proper methods, and automate repetitive tasks efficiently.
These topics are not just theoretical; they’re practical skills that you’ll use in real-world coding scenarios and, importantly, will also be tested in the final WGU D286 OA. Whether you’re iterating through an array to find the largest number, using setters to validate input, or crafting loops to solve complex problems, mastering these concepts will set you up for success.
Remember, practice makes perfect! Try implementing these ideas in small projects to solidify your understanding. Build something fun, like a program to manage a shopping list or calculate factorials, and watch your skills grow. And don’t shy away from reviewing the examples and exercises we covered here—they’ll help you ace those tricky WGU D286 OA questions.
Good luck as you tackle the challenges ahead! With these tools in hand and your determination, there’s no Java problem you can’t solve. Happy coding, and here’s to your success in the WGU D286 module!
