WGU D286 OA Study Guide I - 2025 | Ultimate Guide to Java Constructors, Methods, and Cloud Securityđź“–
Ready to step into the Java universe where you are not simply typing code – you are creating solutions, and impactful applications, and securing them. In this article, we’ll dive into three essential topics that every Java developer needs to know: constructors, methods and cloud security, AWS Shield, and GuardDuty. These concepts will not only help you become a better programmer but also prepare you for WGU D286 OA questions.
First, let’s talk about constructors. Think of them as the starting point for any Java object. Like assembling the pieces of a LEGO set, constructors initialize your objects and get them ready for action. Overloaded constructors give you flexibility, letting you build objects in different ways depending on your needs.
Next, we’ll explore methods. Methods are like the tools and gadgets in your coding toolbox. They perform tasks, solve problems, and make your programs dynamic and reusable. Whether it’s a simple calculation or complex logic, methods let your Java creations come to life.
Finally, there’s security. Visualize your application as having walls that would make up a fortress. AWS Shield and GuardDuty are the defenders that protect it from malicious attacks and suspicious activity. Here you will find information on how these cloud security services work with Java to protect your programs.
But at the end of this article, you will be equipped with the knowledge to build, fine-tune, and protect Java applications without hesitation. Ready to dive in? Let’s get started!
How to Use This Guide for the WGU D286 OA Exam?đź“–
The D286 Java Fundamentals OA exam at WGU evaluates your understanding of Java programming concepts, method usage, and constructors. This guide simplifies the key concepts of Java constructors and overloaded constructors, Java AWS Shield & Guard Duty, and using methods and calling them to help you grasp the topics tested in the exam.
We also provide exam-style questions and practical applications to ensure you’re fully prepared for the questions on the WGU D286 OA exam
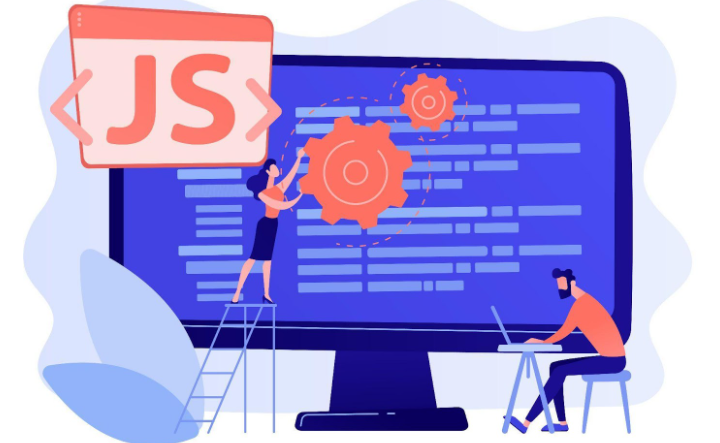
Understanding Java Constructors and Overloaded Constructors For D286 OA📝
In this section, the author will take the readers through one of the most basic topics in Java programming which is constructors and overloaded constructors. Apart from being important for your grasp of OOP, this topic also underpins a myriad of questions within the WGU D286 OA module. Let’s dive in step by step.
What is a Constructor?
A constructor is like setting up the foundation of a building. It is a special method in a Java class that gets triggered automatically when you create an object. Its purpose is to initialize the object’s attributes, ensuring that it begins in a valid state. Think of it as setting the groundwork for your object to function correctly. For example, if you’re designing a robot, the constructor might ensure it starts with working sensors and motors.
Key Points about Constructors:
- Special Method: Unlike regular methods, constructors share the exact name of the class they belong to.
- No Return Type: They do not return any value, not even void.
- Automatic Call: A constructor is invoked automatically when an object is created using the new keyword.
Here’s a simple example of a constructor in action:
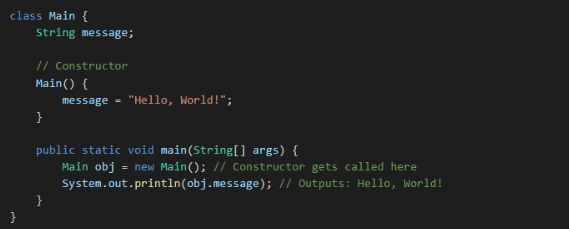
Types of Constructors
Java supports different types of constructors:
1. Default Constructor: If you do not define any constructor in your class, Java automatically provides a default constructor. This constructor initializes the object’s attributes with default values, such as 0 for numbers, false for booleans, and null for objects. It’s like Java giving your object a blank slate to start with, ensuring it has valid, predictable values right from the beginning.
Example:
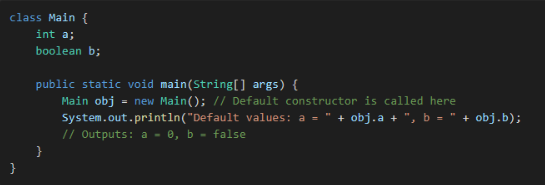
When a class relies on the default constructor, it’s particularly useful in scenarios where you need quick object creation without worrying about custom values initially. However, you might need other types of constructors for more specific initializations.
2. Parameterized Constructor: A parameterized constructor allows you to pass specific values during object creation. This is especially possible when you need a certain object to have certain attributes at its creation time rather than the general ones. For instance, while developing a Student class, a parameterized constructor can provide the real name and age of the student at the time of creating an actual object of the Student class in a single shot, thus being summarized, meaningful, and concise. This is true because objects in real-life applications commonly require parameterized initialization.
Example:
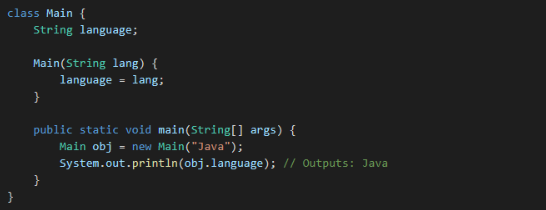
What is Constructor Overloading?
Constructor overloading is very much like allowing your class to do more than one job at a time. It permits you to declare more than one constructor in the same class and with different parameters. This flexibility helps the object to be initialized in a number of ways based on the circumstances. For instance, a Rectangle object might be created with default dimensions, a square dimension, or custom dimensions, all based on which constructor is invoked. Java intelligently determines which constructor to use based on the number and type of arguments passed during object creation, making your code both versatile and efficient.
Here’s an example:
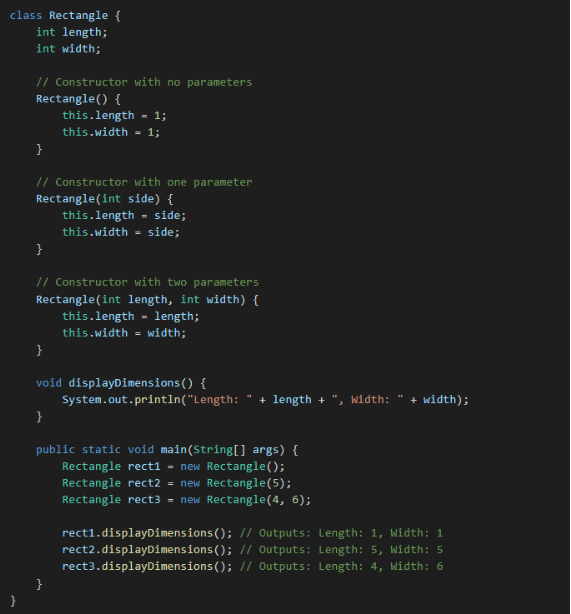
Why Use Constructors?
Constructors serve as the foundation of object initialization. Here are some reasons why they are vital:
- Object Initialization: Ensures that every object starts with a valid state.
- Code Clarity: Reduces redundant code by automating initialization tasks.
- Encapsulation: Promotes encapsulation by controlling how objects are initialized and used.
For instance, imagine an app that tracks user profiles. By using constructors, you can ensure every profile starts with a name and age:
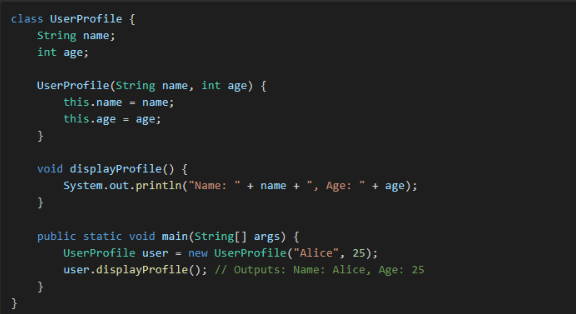
Best Practices for Using Constructors
- Use Descriptive Parameter Names: Helps make your code more readable.
- Avoid Overloading Too Much: Stick to a few meaningful constructor variations.
- Leverage the this Keyword: Use this to avoid ambiguity between instance variables and parameters.
By mastering constructors and overloaded constructors, you’ll build a solid foundation for Java programming, a key area in WGU D286 OA questions. Regular practice with creating and using constructors will ensure success in both your coursework and coding projects.
Next up: Let’s dive into another exciting topic, Java AWS Shield and Guard Duty, which bridges programming and cloud security!
Understanding Java Methods and How to Call Them For D286 OA📝
In this section, we will learn about one of the most fundamental building blocks of Java programming—methods. A proper understanding of methods is essential for writing organized, reusable, and efficient code. This topic also forms a significant portion of the WGU D286 OA questions, so let’s explore it step by step.
What is a Method in Java?
A method is a block of code that performs a specific task when called. Think of it like a recipe: once you have the recipe, you can reuse it to make the same dish over and over again without rewriting the steps.
Key Points about Methods:
- Encapsulation: Methods help encapsulate functionality, keeping your code clean and organized.
- Reusability: Once written, a method can be called multiple times, reducing redundancy.
- Ease of Maintenance: Since methods are independent blocks, making changes to one method doesn’t affect the rest of your program.
Here is a simple example of a method:
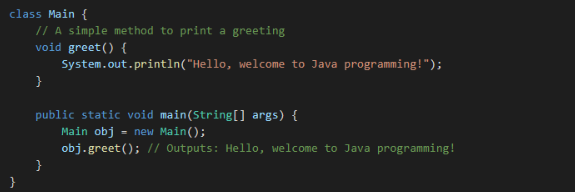
Types of Methods
Java provides flexibility by offering different types of methods to suit your program’s needs:
1. Void Methods: These methods do not return any value. They perform an action and then terminate.
Example:

2. Return Methods: These methods return a value to the caller using the return statement.
Example:
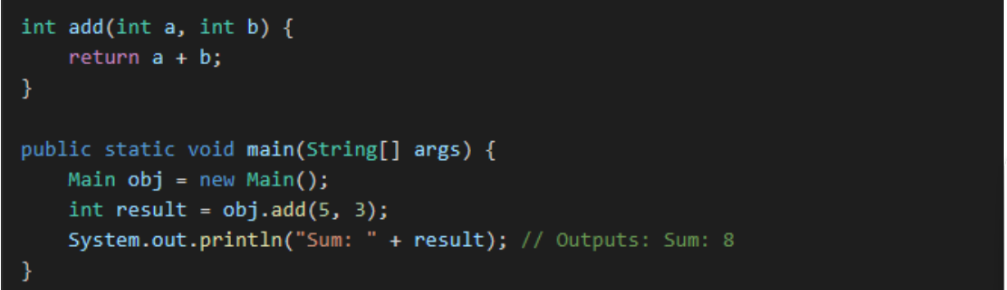
3. Static Methods: Static methods belong to the class and can be called without creating an object of the class.
Example:
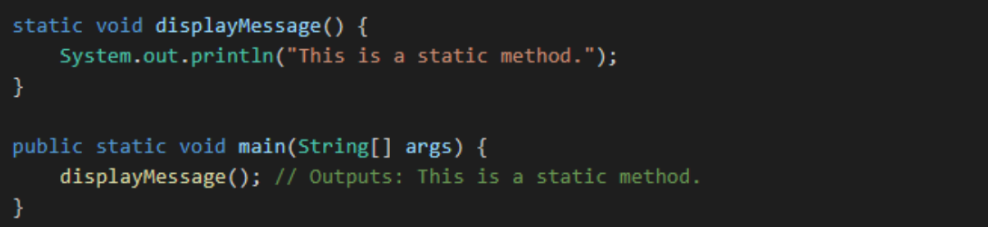
4. Instance Methods: Instance methods require an object of the class to be invoked.
Example:
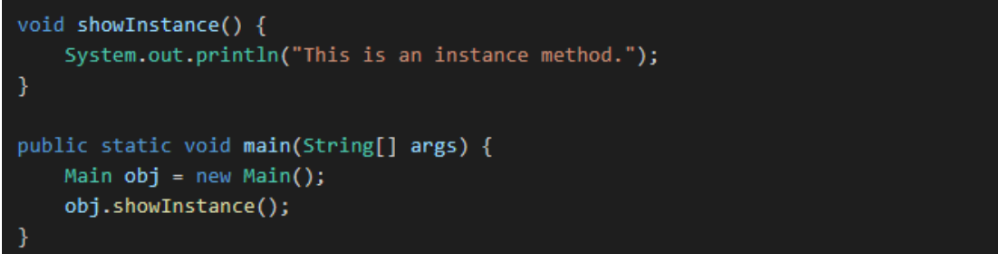
Method Parameters and Arguments
Methods often require inputs to perform their tasks. These inputs are called parameters in the method definition and arguments when values are passed to the method during a call.
Example:
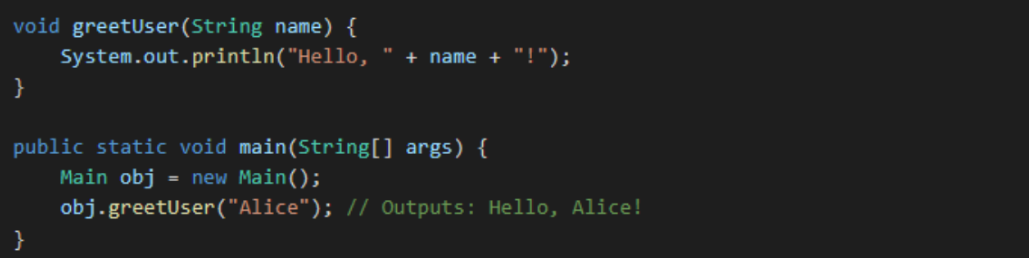
Calling a Method
Calling a method is as simple as using its name followed by parentheses. If the method has parameters, you include the arguments inside the parentheses.
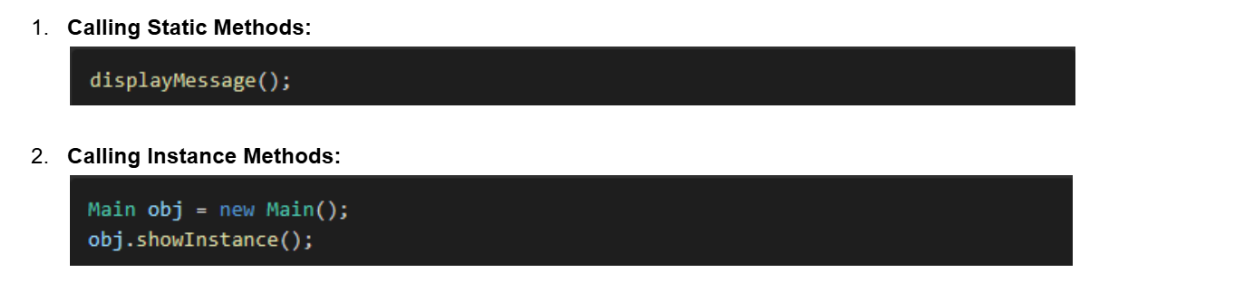
Return Values
When a method returns a value, you can store it in a variable or use it directly in an expression.
Example:
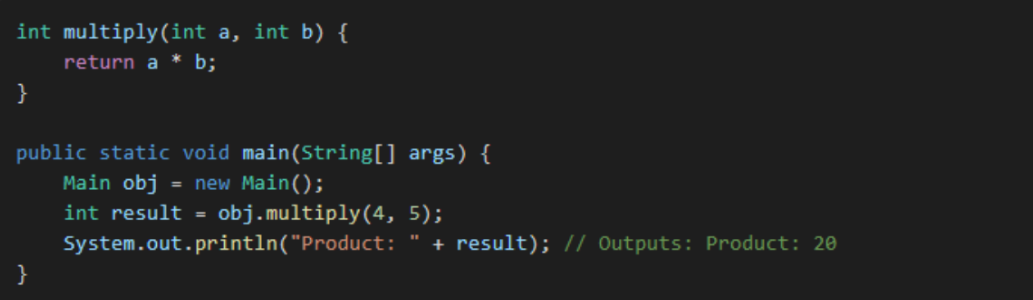
Method Overloading
Method overloading allows you to define multiple methods with the same name but different parameter lists. It is useful for performing similar tasks with different types or numbers of inputs.
Example:
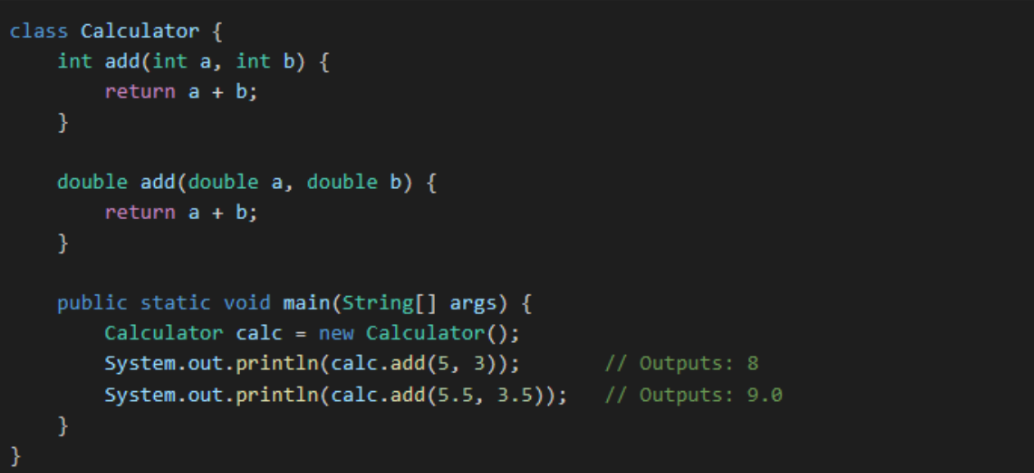
Best Practices
- Use Descriptive Names: Method names should clearly describe their purpose.
- Keep Methods Short: A method should perform a single task to enhance readability.
- Document Methods: Use comments to explain what the method does, especially for complex logic.
- Avoid Too Many Parameters: Keep parameter lists short for better usability.
By mastering methods in Java, you will unlock the ability to write modular, efficient, and reusable code, which is essential for excelling in the WGU D286 OA module. Up next, we will explore how Java AWS Shield and Guard Duty integrate cloud security with programming concepts!
Tired of reading blog articles?
Let’s Watch Our Free WGU D286 Practice Questions Video Below!
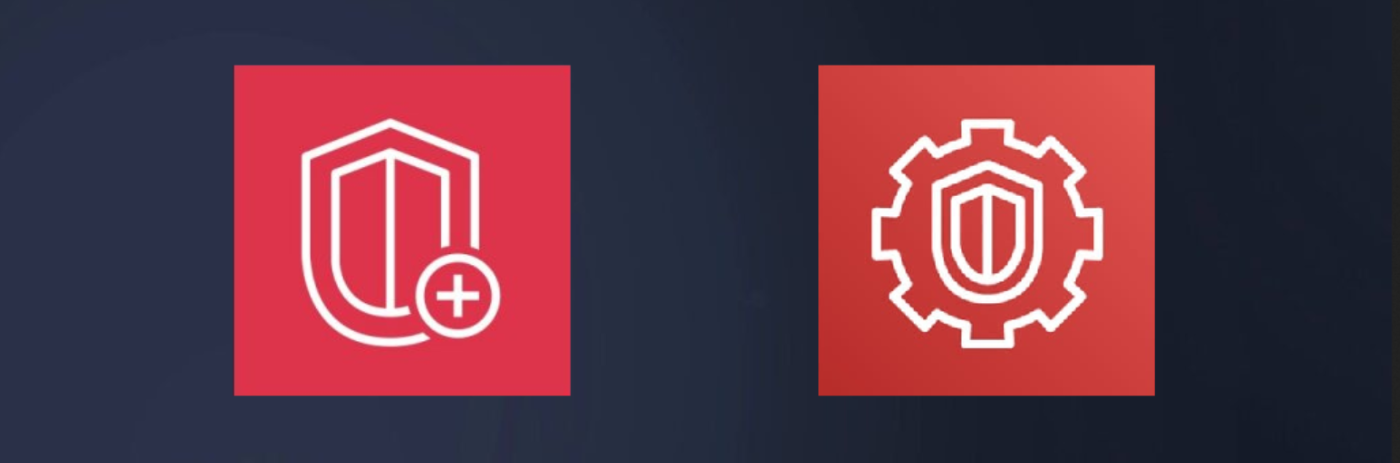
Understanding Java AWS Shield and GuardDuty For D286 OA📝
In this section, we will explore how AWS Shield and AWS GuardDuty contribute to cloud security and how Java developers can integrate these services into their applications. These tools are invaluable for protecting your applications against cyber threats and ensuring a secure cloud environment. Let’s dive into these concepts step by step.
What is AWS Shield?
AWS Shield is a managed service designed to protect applications running on AWS from Distributed Denial of Service (DDoS) attacks. Think of it as a security guard for your cloud resources, always on duty to defend against malicious traffic.
Types of AWS Shield:
- AWS Shield Standard:
- Free for All AWS Customers: Automatically enabled at no additional cost.
- Protection Level: Defends against common infrastructure layer attacks (Layer 3 and 4), such as SYN/UDP floods.
- Key Features:
- Continuous traffic monitoring.
- Automated detection and mitigation of known attack patterns.
- Works seamlessly with other AWS services like CloudFront and Route 53.
- AWS Shield Advanced:
- Paid Service with Enhanced Features: Offers protection against sophisticated DDoS attacks, including application layer attacks (Layer 7).
- Key Features:
- Real-time attack visibility.
- Access to AWS DDoS Response Team (DRT).
- Cost protection for spikes in AWS usage caused by attacks.
- Historical attack data for up to 13 months.
How AWS Shield Works:
- Detection Mechanisms: Continuously monitors network traffic to identify abnormal patterns indicative of DDoS attacks. For example, if a large volume of traffic is directed toward your application unexpectedly, AWS Shield will flag it as a potential attack.
- Automatic Mitigation: Blocks malicious traffic while allowing legitimate requests to pass through, ensuring uninterrupted service.
- Integration with AWS Services: AWS Shield integrates with services like AWS WAF (Web Application Firewall) to provide additional protection against Layer 7 attacks.
Example: Mitigation in Action
Suppose an attacker launches a SYN flood attack against your application. AWS Shield detects unusual traffic patterns and deploys pre-configured rules to filter out malicious packets while allowing legitimate users to access the service without interruption.
What is AWS GuardDuty?
AWS GuardDuty is a threat detection service that continuously monitors your AWS environment for suspicious activity and unauthorized access. Imagine it as a detective keeping watch over your cloud infrastructure.
Key Features of AWS GuardDuty:
- Threat Intelligence: Uses data from AWS, third-party sources, and machine learning to detect potential threats, such as compromised instances or anomalous behavior.
- Anomaly Detection: Identifies unusual account activity, such as unauthorized API calls or instances performing actions outside their normal behavior.
- Seamless Integration: Works with AWS Lambda for automated responses to detected threats, enabling real-time remediation.
- Global Threat Dashboard: Provides a bird’s-eye view of potential threats across AWS environments, helping users stay informed about global attack trends.
Real-Time Monitoring and Visibility
One of the standout features of AWS Shield Advanced is its real-time monitoring capability. Users receive instant notifications via Amazon CloudWatch when a potential attack is detected. This allows developers and system administrators to respond promptly, minimizing downtime and ensuring service continuity.
Similarly, AWS GuardDuty provides detailed alerts whenever it detects unauthorized behavior, such as:
- Unexpected changes in IAM roles.
- Unauthorized access to S3 buckets.
- Abnormal data transfer volumes.
Integrating AWS Services with Java Applications
Java developers can use the AWS SDK (Software Development Kit) to integrate AWS Shield and GuardDuty into their applications. This enables your Java programs to monitor and respond to potential security threats.
Example: Connecting to AWS Services Using Java SDK
Here’s how you can use the AWS SDK to interact with AWS services:
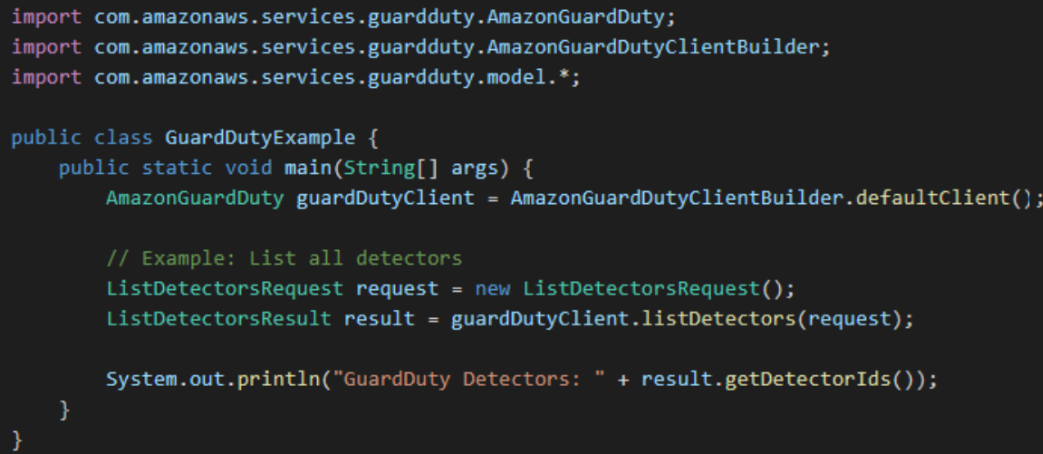
Practical Use Cases:
- DDoS Protection: Use AWS Shield to safeguard APIs and web applications by automatically mitigating attacks without manual intervention.
- Threat Detection: Implement GuardDuty to monitor AWS accounts and flag suspicious behavior such as unusual API requests or data access patterns.
- Automated Response: Combine AWS GuardDuty with AWS Lambda to create automated workflows for responding to security incidents. For example, you can configure a Lambda function to block suspicious IP addresses as soon as GuardDuty raises an alert.
Best Practices for Cloud Security
- Enable Multi-Layered Protection: Combine AWS Shield, GuardDuty, AWS WAF, and IAM policies for comprehensive security.
- Monitor Regularly: Set up Amazon CloudWatch alerts to track security events in real-time.
- Automate Responses: Use AWS Lambda to automate incident responses, such as isolating compromised instances or notifying administrators.
- Leverage Historical Data: Use historical attack data from AWS Shield Advanced to analyze patterns and improve defenses.
- Apply the Principle of Least Privilege: Limit access to AWS resources using IAM policies to reduce the risk of unauthorized actions.
Importance For D286 OA
Understanding AWS Shield and GuardDuty is not just about cloud security; it’s also about integrating security measures directly into your Java applications. By leveraging these tools, Java developers can:
- Ensure their applications remain available even during cyberattacks.
- Detect and respond to threats in real-time.
- Build user trust by demonstrating a commitment to robust security practices.
By leveraging AWS Shield and GuardDuty, Java developers can build robust, secure applications while ensuring a safe cloud environment. These tools are essential for addressing cybersecurity challenges and are a significant focus area in WGU D286 OA questions. Stay tuned as we continue exploring more exciting topics in Java programming!
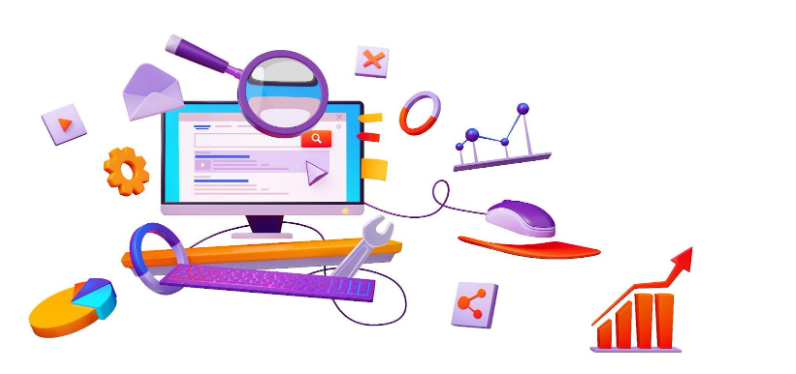
Mastering Java for WGU D286 OA Successđź“„
Congratulations on exploring Java constructors, methods, and cloud security! These topics are not just concepts—they’re the foundation for creating efficient, secure, and powerful applications. More importantly, they will play a key role in your WGU D286 OA final assessment.
Constructors are the backbone of object creation, methods breathe life into your code, and AWS Shield and GuardDuty protect your applications in a cloud environment. Together, they equip you with the skills to excel both in assessments and real-world challenges.
As you prepare for the final OA, practice creating constructors, designing methods, and integrating cloud security. Use resources like practice tests and the AWS SDK to refine your understanding. Keep coding, stay curious, and embrace the journey of learning.
Good luck with your WGU D286 OA—you’ve got this!
